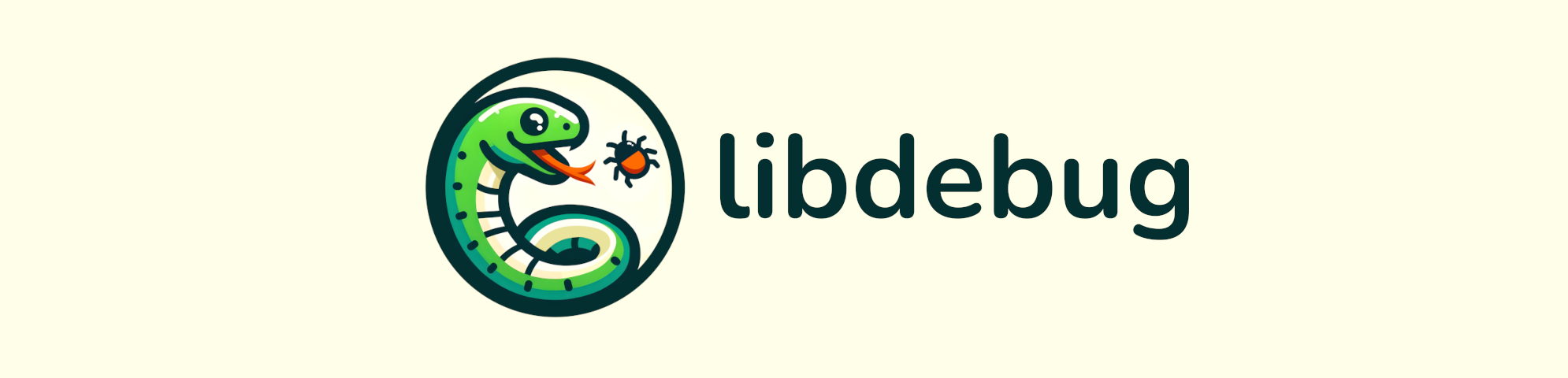
Quick Start#
Welcome to libdebug! This powerful Python library can be used to debug your binary executables programmatically, providing a robust, user-friendly interface.
Debugging multithreaded applications can be a nightmare, but libdebug has you covered. Hijack, and manage signals and syscalls with a simple API.
Supported Architectures#
libdebug currently supports Linux under the x86_64 architecture.
Other operating systems and architectures are not supported at this time.
Dependencies#
To install libdebug, you first need to have some dependencies that will not be automatically resolved. Depending on your distro, their names may change.
sudo apt install -y python3 python3-dev libdwarf-dev libelf-dev libiberty-dev linux-headers-generic libc6-dbg
sudo apt install -y python3 python3-dev libdwarf-dev libelf-dev libiberty-dev linux-headers-generic libc6-dbg
sudo pacman -S python libelf libdwarf gcc make debuginfod
sudo dnf install -y python3 python3-devel kernel-devel binutils-devel libdwarf-devel
Installation#
Installing libdebug once you have dependencies is as simple as running the following command:
python3 -m pip install libdebug
This will install the stable build of the library. If you don’t mind a few hiccups, you can install the latest dev build from the Github repository:
python3 -m pip install git+https://github.com/libdebug/libdebug.git@dev
Your first script#
Now that you have libdebug installed, you can start using it in your scripts. Here is a simple example of how to use libdebug to debug a binary:
from libdebug import debugger
d = debugger("./test")
# Start debugging from the entry point
d.run()
my_breakpoint = d.breakpoint("function")
# Continue the execution until the breakpoint is hit
d.cont()
# Print RAX
print(f"RAX is {hex(d.regs.rax)}")
# Kill the process
d.kill()
The above script will run the binary test in the working directory and stop at the function corresponding to the symbol “function”. It will then print the value of the RAX register and kill the process.
Contents: